================ Start Lecture #11
================
3.4.3: Recovery from deadlock
Preemption
Perhaps you can temporarily preempt a resource from a process. Not
likely.
Rollback
Database (and other) systems take periodic checkpoints. If the
system does take checkpoints, one can roll back to a checkpoint
whenever a deadlock is detected. Somehow must guarantee forward
progress.
Kill processes
Can always be done but might be painful. For example some
processes have had effects that can't be simply undone. Print, launch
a missile, etc.
Remark:
We are doing 3.6 before 3.5 since 3.6 is easier.
3.6: Deadlock Prevention
Attack one of the coffman/havender conditions
3.6.1: Attacking Mutual Exclusion
Idea is to use spooling instead of mutual exclusion. Not
possible for many kinds of resources
3.6.2: Attacking Hold and Wait
Require each processes to request all resources at the beginning
of the run. This is often called One Shot.
3.6.3: Attacking No Preempt
Normally not possible.
3.6.4: Attacking Circular Wait
Establish a fixed ordering of the resources and require that they
be requested in this order. So if a process holds resources #34 and
#54, it can request only resources #55 and higher.
It is easy to see that a cycle is no longer possible.
Homework: 7.
3.5: Deadlock Avoidance
Let's see if we can tiptoe through the tulips and avoid deadlock
states even though our system does permit all four of the necessary
conditions for deadlock.
An optimistic resource manager is one that grants every
request as soon as it can. To avoid deadlocks with all four
conditions present, the manager must be smart not optimistic.
3.5.1 Resource Trajectories
We plot progress of each process along an axis.
In the example we show, there are two processes, hence two axes, i.e.,
planar.
This procedure assumes that we know the entire request and release
pattern of the processes in advance so it is not a practical
solution.
I present it as it is some motivation for the practical solution that
follows, the Banker's Algorithm.
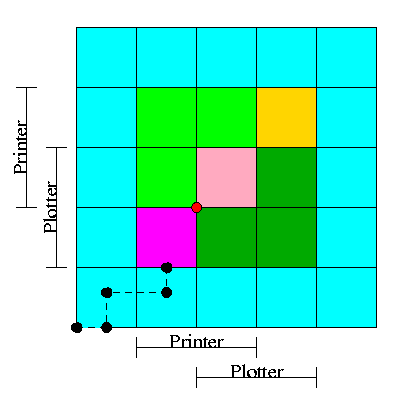
- We have two processes H (horizontal) and V.
- The origin represents them both starting.
- Their combined state is a point on the graph.
- The parts where the printer and plotter are needed by each process
are indicated.
- The dark green is where both processes have the plotter and hence
execution cannot reach this point.
- Light green represents both having the printer; also impossible.
- Pink is both having both printer and plotter; impossible.
- Gold is possible (H has plotter, V has printer), but you can't get
there.
- The upper right corner is the goal; both processes have finished.
- The red dot is ... (cymbals) deadlock. We don't want to go there.
- The cyan is safe. From anywhere in the cyan we have horizontal
and vertical moves to the finish point (the upper right corner)
without hitting any impossible area.
- The magenta interior is very interesting. It is
- Possible: each processor has a different resource
- Not deadlocked: each processor can move within the magenta
- Deadly: deadlock is unavoidable. You will hit a magenta-green
boundary and then will no choice but to turn and go to the red dot.
- The cyan-magenta border is the danger zone.
- The dashed line represents a possible execution pattern.
- With a uniprocessor no diagonals are possible. We either move to
the right meaning H is executing or move up indicating V is executing.
- The trajectory shown represents.
- H excuting a little.
- V excuting a little.
- H executes; requests the printer; gets it; executes some more.
- V executes; requests the plotter.
- The crisis is at hand!
- If the resource manager gives V the plotter, the magenta has been
entered and all is lost. ``Abandon all hope ye who enter here''
--Dante.
- The right thing to do is to deny the request, let H execute moving
horizontally under the magenta and dark green. At the end of the dark
green, no danger remains, both processes will complete successfully.
Victory!
- This procedure is not practical for a general purpose OS since it
requires knowing the programs in advance. That is, the resource
manager, knows in advance what requests each process will make and in
what order.
Homework: 10, 11, 12.
3.5.2: Safe States
Avoiding deadlocks given some extra knowledge.
- Not surprisingly, the resource manager knows how many units of each
resource it had to begin with.
- Also it knows how many units of each resource it has given to
each process.
- It would be great to see all the programs in advance and thus know
all future requests, but that is asking for too much.
- Instead, when each process when it starts, it gives its maximum usage.
That is each process at startup states, for each resource, the maximum
number of units it can possibly ask for.
This is called the claim of the process.
- If during the run the process asks for more than its claim,
abort it.
- If it claims more than it needs, the result is that the
resource manager will be more conservative than need be and there
will be more waiting.
Definition: A state is safe
if there is an ordering of the processes such that: if the
processes are run in this order, they will all terminate (assuming
none exceeds its claim).
Give an example of all four possibilities. A state that is
- Safe and deadlocked--not possible
- Safe and not deadlocked
- Not safe and deadlocked
- Not safe and not deadlocked--interesting
A manager can determine if a state is safe.
- Since the manager know all the claims, it can determine the maximum
amount of additional resources each process can request.
- The manager knows how many units of each resource it has left.
The manager then follows the following procedure, which is part of
Banker's Algorithms discovered by Dijkstra, to
determine if the state is safe.
- If there are no processes remaining, the state is
safe.
- Seek a process P whose max additional requests is less than
what remains (for each resource type).
- If no such process can be found, then the state is
not safe.
- The banker (manager) knows that if it refuses all requests
excepts those from P, then it will be able to satisfy all
of P's requests. Why?
Ans: Look at how P was chosen.
- The banker now pretends that P has terminated (since the banker
knows that it can guarantee this will happen). Hence the banker
pretends that all of P's currently held resources are returned. This
makes the banker richer and hence perhaps a process that was not
eligible to be chosen as P previously, can now be chosen.
- Repeat these steps.
Example 1
A safe state with 22 units of one resource
process | claim | current | max need |
X | 3 | 1 | 2 |
Y | 11 | 5 | 6 |
Z | 19 | 10 | 9 |
Total | 16 |
Available | 6 |
- One resource type R with 22 unit
- Three processes X, Y, and Z with claims 3, 11, and 19 respectively.
- Currently the processes have 1, 5, and 10 units respectively.
- Hence the manager currently has 6 units left.
- Also note that the max additional needs for processes are 2, 6, 9
- So the manager cannot assure (with its current
remaining supply of 6 units) that Z can terminate. But that is
not the question.
- This state is safe
- Use 2 units to satisfy X; now the manager has 7 units.
- Use 6 units to satisfy Y; now the manager has 12 units.
- Use 9 units to satisfy Z; done!
Example 2
A unsafe state with 22 units of one resource
process | claim | current | max need |
X | 3 | 1 | 2 |
Y | 11 | 5 | 6 |
Z | 19 | 12 | 7 |
Total | 18 |
Available | 4 |
Start with example 1 and assume that Z now requests 2 units and we
grant them.
- Currently the processes have 1, 5, and 12 units respectively.
- The manager has 4 units.
- The max additional needs are 2, 6, and 7.
- This state is unsafe
- Use 2 unit to satisfy X; now the manager has 5 units.
- Y needs 6 and Z needs 7 so we can't guarantee satisfying either
- Note that we were able to find a process that can terminate (X)
but then we were stuck. So it is not enough to find one process.
Must find a sequence of all the processes.