================ Start Lecture #24
================
Chapter 6: Deadlocks
A deadlock occurs when a every member of a set of
processes is waiting for an event that can only be caused
by a member of the set.
Often the event waited for is the release of a resource.
In the automotive world deadlocks are called gridlocks.
- The processes are the cars.
- The resources are the spaces occupied by the cars
Reward: One point extra credit on the final exam
for anyone who brings a real (e.g., newspaper) picture of an
automotive deadlock. You must bring the clipping to the final and it
must be in good condition. Hand it in with your exam paper.
For a computer science example consider two processes A and B that
each want to print a file currently on tape.
- A has obtained ownership of the printer and will release it after
printing one file.
- B has obtained ownership of the tape drive and will release it after
reading one file.
- A tries to get ownership of the tape drive, but is told to wait
for B to release it.
- B tries to get ownership of the printer, but is told to wait for
A to release the printer.
Bingo: deadlock!
6.1: Resources:
The resource is the object granted to a process.
- Resources come in two types
- Preemptable, meaning that the resource can be
taken away from its current owner (and given back later). An
example is memory.
- Non-preemptable, meaning that the resource
cannot be taken away. An example is a printer.
- The interesting issues arise with non-preemptable resources so
those are the ones we study.
- Life history of a resource is a sequence of
- Request
- Allocate
- Use
- Release
- Processes make requests, use the resourse, and release the
resourse. The allocate decisions are made by the system and we will
study policies used to make these decisions.
6.2: Deadlocks
To repeat: A deadlock occurs when a every member of a set of
processes is waiting for an event that can only be caused
by a member of the set.
Often the event waited for is the release of
a resource.
6.2.1: (Necessary) Conditions for Deadlock
The following four conditions (Coffman; Havender) are
necessary but not sufficient for deadlock. Repeat:
They are not sufficient.
- Mutual exclusion: A resource can be assigned to at most one
process at a time (no sharing).
- Hold and wait: A processing holding a resource is permitted to
request another.
- No preemption: A process must release its resources; they cannot
be taken away.
- Circular wait: There must be a chain of processes such that each
member of the chain is waiting for a resource held by the next member
of the chain.
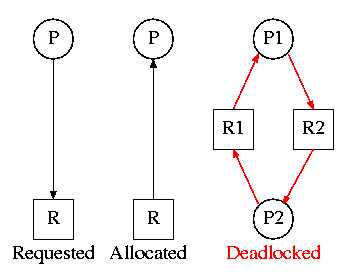
6.2.2: Deadlock Modeling
On the right is the Resource Allocation Graph,
also called the Reusable Resource Graph.
- The processes are circles.
- The resources are squares.
- An arc (directed line) from a process P to a resource R signifies
that process P has requested (but not yet been allocated) resource R.
- An arc from a resource R to a process P indicates that process P
has been allocated resource R.
Homework: 1.
Consider two concurrent processes P1 and P2 whose programs are.
P1: request R1 P2: request R2
request R2 request R1
release R2 release R1
release R1 release R2
On the board draw the resource allocation graph for various possible
executions of the processes, indicating when deadlock occurs and when
deadlock is no longer avoidable.
There are four strategies used for dealing with deadlocks.
- Ignore the problem
- Detect deadlocks and recover from them
- Avoid deadlocks by carefully deciding when to allocate resources.
- Prevent deadlocks by violating one of the 4 necessary conditions.
6.3: Ignoring the problem--The Ostrich Algorithm
The ``put your head in the sand approach''.
- If the likelihood of a deadlock is sufficiently small and the cost
of avoiding a deadlock is sufficiently high it might be better to
ignore the problem. For example if each PC deadlocks once per 100
years, the one reboot may be less painful that the restrictions needed
to prevent it.
- Clearly not a good philosophy for nuclear missile launchers.
- For embedded systems (e.g., missile launchers) the programs run
are fixed in advance so many of the questions Tanenbaum raises (such
as many processes wanting to fork at the same time) don't occur.
6.4: Detecting Deadlocks and Recovering from them
6.4.1: Detecting Deadlocks with single unit resources
Consider the case in which there is only one
instance of each resource.
- So a request can be satisfied by only one specific resource.
- In this case the 4 necessary conditions for
deadlock are also sufficient.
- Remember we are making an assumption (single unit resources) that
is often invalid. For example, many systems have several printers and
a request is given for ``a printer'' not a specific printer.
Similarly, one can have many tape drives.
- So the problem comes down to finding a directed cycle in the resource
allocation graph. Why?
Answer: Because the other three conditions are either satisfied by the
system we are studying or are not in which case deadlock is not a
question. That is, conditions 1,2,3 are conditions on the system in
general not on what is happening right now.
To find a directed cycle in a directed graph is not hard. The
algorithm is in the book. The idea is simple.
- For each node in the graph do a depth first traversal (hoping the
graph is a DAG (directed acyclic graph), building a list as you go
down the DAG.
- If you ever find the same node twice on your list, you have found
a directed cycle and the graph is not a DAG and deadlock exists among
the processes in your current list.
- If you never find the same node twice, the graph is a DAG and no
deadlock occurs.
- The searches are finite since the list size is bounded by the
number of nodes.
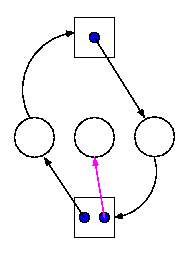
6.4.2: Detecting Deadlocks with multiple unit resources
This is more difficult.
- The figure on the right shows a resource allocation graph with
multiple unit resources.
- Each unit is represented by a dot in the box.
- Request edges are drawn to the box since they represent a request
for any dot in the box.
- Allocation edges are drawn from the dot to represent that this
unit of the resource has been assigned (but all units of a resource
are equivalent and the choice of which one to assign is arbitrary).
- Note that there is a directed cycle in black, but there is no
deadlock. Indeed the middle process might finish, erasing the magenta
arc and permitting the blue dot to satisfy the rightmost process.
- The book gives an algorithm for detecting deadlocks in this more
general setting. The idea is as follows.
- look fora process that might be able to terminate (i.e., all
its request arcs can be satisfied).
- If one is found pretend that it does terminate (erase all its
arcs), and repeat step 1.
- If any processes remain, they are deadlocked.
- We will soon do in detail an algorithm (the Banker's algorithm) that
has some of this flavor.
6.4.3: Recovery from deadlock
Preemption
Perhaps you can temporarily preempt a resource from a process. Not
likely.
Rollback
Database (and other) systems take periodic checkpoints. If the
system does take checkpoints, one can roll back to a checkpoint
whenever a deadlock is detected. Somehow must guarantee forward
progress.
Kill processes
Can always be done but might be painful. For example some
processes have had effects that can't be simply undone. Print, launch
a missile, etc.
Remark:
We are doing 6.6 before 6.5 since 6.6 is easier.
6.6: Deadlock Prevention
Attack one of the coffman/havender conditions
6.6.1: Attacking Mutual Exclusion
Idea is to use spooling instead of mutual exclusion. Not
possible for many kinds of resources
6.6.2: Attacking Hold and Wait
Require each processes to request all resources at the beginning
of the run. This is often called One Shot.
6.6.3: Attacking No Preempt
Normally not possible.
6.6.4: Attacking Circular Wait
Establish a fixed ordering of the resources and require that they
be requested in this order. So if a process holds resources #34 and
#54, it can request only resources #55 and higher.
It is easy to see that a cycle is no longer possible.
6.5: Deadlock Avoidance
Let's see if we can tiptoe through the tulips and avoid deadlock
states even though our system does permit all four of the necessary
conditions for deadlock.
An optimistic resource manager is one that grants every
request as soon as it can. To avoid deadlocks with all four
conditions present, the manager must be smart not optimistic.
6.5.1 Resource Trajectories
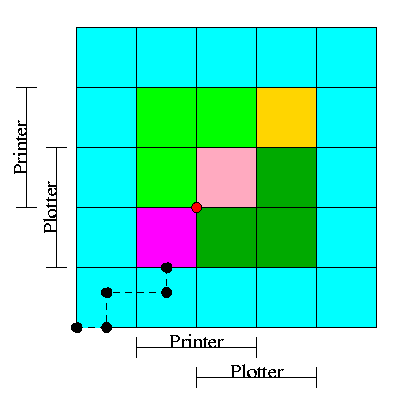
- We have two processes H (horizontal) and V.
- The origin represents them both starting.
- Their combined state is a point on the graph.
- The parts where the printer and plotter are needed by each process
are indicated.
- The dark green is where both processes have the plotter and hence
execution cannot reach this point.
- Light green represents both having the printer; also impossible.
- Pink is both having both printer and plotter; impossible.
- Gold is possible (H has plotter, V has printer), but you can't get
there.
- The upper right corner is the goal both processes finished.
- The red dot is ... (cymbals) deadlock. We don't want to go there.
- The cyan is safe. From anywhere in the cyan we have horizontal
and vertical moves to the finish line without hitting any impossible
area.
- The magenta interior is very interesting. It is
- Possible: each processor has a different resource
- Not deadlocked: each processor can move within the magenta
- Deadly: deadlock is unavoidable. You will hit a magenta-green
boundary and then will no choice but to turn and go to the red dot.
- The cyan-magenta border is the danger zone
- The dashed line represents a possible execution pattern.
- With a uniprocessor no diagonals are possible. We either move to
the right meaning H is executing or move up indicating V.
- The trajectory shown represents.
- H excuting a little.
- V excuting a little.
- H executes; requests the printer; gets it; executes some more.
- V executes; requests the plotter.
- The crisis is at hand!
- If the resource manager gives V the plotter, the magenta has been
entered and all is lost. ``Abandon all hope yee who enter here''
--Dante.
- The right thing to do is to deny the request, let H execute moving
horizontally under the magenta and dark green. At the end of the dark
green, no danger remains, both processes will complete successfully.
Victory!
- This procedure is not practical for a general purpose OS since it
requires knowing the programs in advance. That is, the resource
manager, knows in advance what requests each process will make and in
what order.