Computer Architecture
1999-2000 Fall
MW 3:30-4:45
Ciww 109
Allan Gottlieb
gottlieb@nyu.edu
http://allan.ultra.nyu.edu/~gottlieb
715 Broadway, Room 1001
212-998-3344
609-951-2707
email is best
======== START LECTURE #12
========
Midterm exam 25 Oct.
Lab 2. Due 1 November. Extend Modify lab 1 to a 32 bit alu that
in addition handles sub, slt, zero detect, and overflow. That is,
produce a gate level simulation of Figure 4.19. This figure is also
in the class notes; it is the penultimate figure before ``Fast Adders''.
It is NOW DEFINITE that on monday 23 Oct, my office
hours will have to move from 2:30--3:30 to 1:30-2:30 due to a
departmental committee meeting.
Don't forget the mirror site. My main website will be
going down for an OS upgrade at some point. Start at http://cs.nyu.edu
End of Notes:
Chapter 5: The processor: datapath and control
Homework:
Start Reading Chapter 5.
5.1: Introduction
We are going to build the MIPS processor
Figure 5.1 redrawn below shows the main idea
Note that the instruction gives the three register numbers as well
as an immediate value to be added.
- No instruction actually does all this.
- We have datapaths for all possibilities.
- Will see how we arrange for only certain datapaths to be used for
each instruction type.
- For example R type uses all three registers but not the
immediate field.
- The I type uses the immediate but not all three registers.
- The memory address for a load or store is the sum of a register
and an immediate.
- The data value to be stored comes from a register.
5.2: Building a datapath
Let's begin doing the pieces in more detail.
Instruction fetch
We are ignoring branches for now.
- How come no write line for the PC register?
- Ans: We write it every cycle.
- How come no control for the ALU
- Ans: This one always adds
R-type instructions
- ``Read'' and ``Write'' in the diagram are adjectives not verbs.
- The 32-bit bus with the instruction is divided into three 5-bit
buses for each register number (plus other wires not shown).
- Two read ports and one write port, just as we learned in chapter 4.
- The 3-bit control consists of Bnegate and Op from chapter 4.
- The RegWrite control line is always asserted for R-type
instructions.
Homework: What would happen if the RegWrite line
had a stuck-at-0 fault (was always deasserted)?
What would happen if the RegWrite line
had a stuck-at-1 fault (was always asserted)?
load and store
lw $r,disp($s)
sw $r,disp($s)
- lw $r,disp($s):
- Computes the effective address formed by adding the 16-bit
immediate constant ``disp'' to the contents of register $s.
- Fetches the value in data memory at this address.
- Inserts this value into register $r.
- sw $r,disp($s):
- Computes the same effective address as lw $r,disp($s)
- Stores the contents of register $r into this address
- We have a 32-bit adder so need to extend the 16-bit immediate
constant to 32 bits. Produce an additional 16 HOBs all equal to the
sign bit of the 16-bit immediate constant. This is called sign
extending the constant.
- RegWrite is deasserted for sw and asserted for lw.
- MemWrite is asserted for sw and deasserted for lw.
- I don't see the need for MemRead; perhaps it is there for power
saving.
- The ALU Operation is set to add for lw and sw.
- For now we just write down which control lines are asserted and
deasserted. Later we will do the circuit for to calculate the control
lines from the instruction word.
Homework: What would happen if the RegWrite line
had a stuck-at-0 fault (was always deasserted)?
What would happen if the RegWrite line
had a stuck-at-1 fault (was always asserted)?
What would happen if the MemWrite line
had a stuck-at-0 fault (was always deasserted)?
What would happen if the MemWrite line
had a stuck-at-1 fault (was always asserted)?
There is a cheat here.
- For lw we read register r (and read s)
- For sw we write register r (and read s)
- But we indicated that the same bits in the instruction always go to
the same ports in the register file.
- We are ``mux deficient''.
- We will put in the mux later
Branch on equal (beq)
Compare two registers and branch if equal.
- To check for equal we subtract and test for zero (our ALU does
this).
- If $r=$s, the target of the branch beq $r,$s,disp is the sum of
- The program counter PC after it has been incremented,
that is the address of the next sequential instruction
- The 16-bit immediate constant ``disp'' (treated as a signed
number) left shifted 2 bits.
- The value of PC after the increment is available. We computed it
in the basic instruction fetch datapath.
- Since the immediate constant is signed it must be sign extended.
Homework: What would happen if the RegWrite line
had a stuck-at-0 fault (was always deasserted)?
What would happen if the RegWrite line
had a stuck-at-1 fault (was always asserted)?
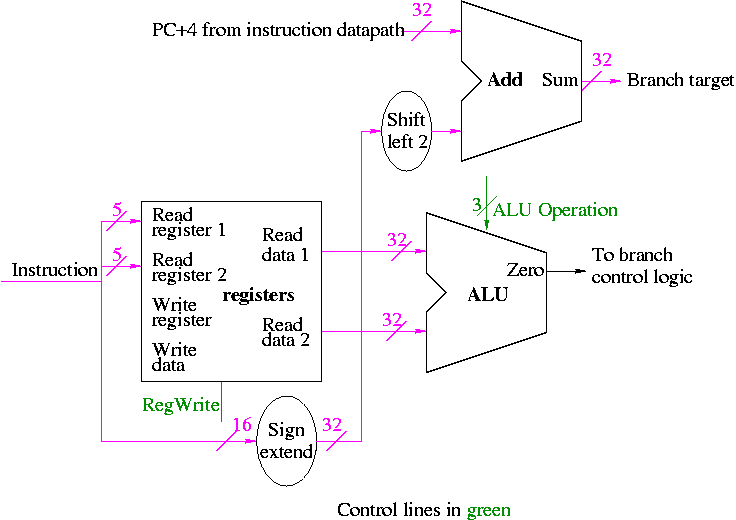
- The top ``alu symbol'' labeled ``add'' is just an adder so does
not need any control
- The shift left 2 is not a shifter. It simply moves wires and
includes two zero wires. We need a 32-bit version. Below is a 5 bit
version.
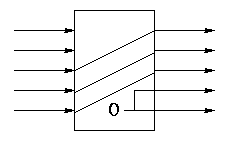
5.3: A simple implementation scheme
We will just put the pieces together and then figure out the control
lines that are needed and how to set them. We are not now worried
about speed.
We are assuming that the instruction memory and data memory are
separate. So we are not permitting self modifying code. We are not
showing how either memory is connected to the outside world (i.e. we
are ignoring I/O).
We have to use the same register file with all the pieces since when a
load changes a register a subsequent R-type instruction must see the
change, when an R-type instruction makes a change the lw/sw must see
it (for loading or calculating the effective address, etc.
We could use separate ALUs for each type but it is easy not to so we
will use the same ALU for all. We do have a separate adder for
incrementing the PC.
Combining R-type and lw/sw
The problem is that some inputs can come from different sources.
- For R-type, both ALU operands are registers. For I-type (lw/sw)
the second operand is the (sign extended) immediate field.
- For R-type, the write data comes from the ALU. For lw it comes
from the memory.
- For R-type, the write register comes from field rd, which is bits
15-11. For sw, the write register comes from field rt, which is bits
20-16.
We will deal with the first two now by using a mux for each. We
will deal with the third shortly by (surprise) using a mux.
Combining R-type and lw/sw
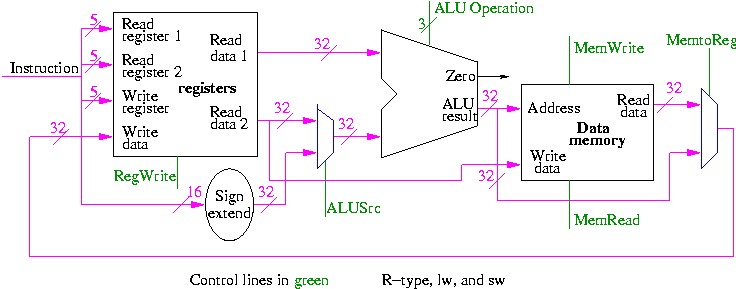