Scripting Languages CSCI-GA.3033.003, Summer 2012 hw10
- Dates:
Assigned Thursday 7/26/2012, due Friday 8/3/2012 at 6pm.
- Topic:
Other scripting languages
- Total points:
40
- Instructions:
Please put your solutions to all questions (including both the
concept questions and the programming questions) in a
single plain-text file, such as what you get when using Emacs, Vi,
Notepad, or the “save as text only” feature in Word.
Your JavaScript code must run in both Mozilla's Firefox
browser and Microsoft's Internet Explorer browser.
If you don't have a computer with these browsers, test your code
on the Windows machines in the
labs
WWH 229 and WWH 230. Please email your solutions to hw10 to the grader.
- Grader for hw10:
Christian Keitel (ck1456@nyu.edu)
Concept Questions
hw10_1 Block parameters in Ruby
Consider the following Ruby script.
#!/usr/bin/env ruby
class Tree
attr_accessor :value, :left, :right
def initialize(value, *leftRight)
@value = value
if 0 < leftRight.length()
@left = leftRight[0]
@right = leftRight[1]
end
end
def preorder()
yield @value
if @left
@left.preorder {|v| yield v }
end
if @right
@right.preorder {|v| yield v }
end
end
end
$my_tree = Tree.new(4,
Tree.new(1, Tree.new(7), Tree.new(3)),
Tree.new(5, Tree.new(6), Tree.new(2)))
def print_preorder(tree)
puts 'preorder:'
tree.preorder {|y| puts y.to_s + "\n" }
end
print_preorder($my_tree)
- 1a.
- (4 points) Predict what the code should print. Then run it.
What does it print?
- 1b.
- (6 points) Add another top-level function print_max
that uses the preorder method to compute the maximum of all
values in the tree. For example, the following call:
print_max($my_tree)
should cause the following output to be printed:
max:
7
hw10_2 Generators in Python
Consider the following Python script.
#!/usr/bin/env python
class Tree:
def __init__(self, value, left=None, right=None):
self.value = value
self.left = left
self.right = right
def preorder(self):
yield self.value
if self.left:
for v in self.left.preorder():
yield v
if self.right:
for v in self.right.preorder():
yield v
my_tree = Tree(4,
Tree(1, Tree(7), Tree(3)),
Tree(5, Tree(6), Tree(2)))
def print_preorder(tree):
print 'preorder:'
for y in my_tree.preorder():
print y
print_preorder(my_tree)
- 2a.
- (4 points) Predict what the code should print. Then run it.
What does it print?
- 2b.
- (6 points) Add another generator method Tree.postorder
that walks the tree in post-order instead of pre-order. A post-order walk
first visits all nodes in the left subtree, then all nodes in the right
subtree, then the current node. The following picture shows a tree for
which a post-order walk would visit nodes in alphabetical
order:
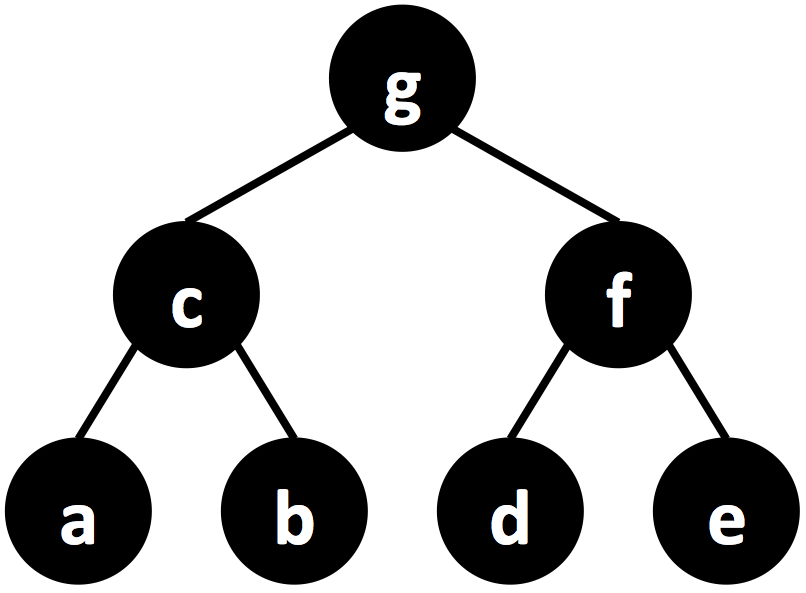
After you write the generator Tree.postorder, the following code:
def print_postorder(tree):
print 'postorder:'
for y in my_tree.postorder():
print y
print_postorder(my_tree)
should cause the following output to be printed:
postorder:
7
3
1
6
2
5
4
hw10_3 Block parameters vs. Coroutines
- 3a.
- (4 points)
Briefly explain the difference between block parameters and coroutines.
- 3b.
- (3 points)
Give an advantage of block parameters compared to coroutines.
- 3c.
- (3 points)
Give an advantage of coroutines compared to block parameters.
Programming exercises
hw10_4 Closures in JavaScript
(10 points) Consider the following skeleton HTML file with JavaScript code:
<html><head>
<meta http-equiv="Content-Script-Type" content="text/javascript" />
<script>
function Tree(value, left, right) {
//implement constructor here
}
Tree.prototype.preorder = function(callback) {
//implement method here
}
</script>
</head><body>
<script>
myTree = new Tree(4,
new Tree(1, new Tree(7), new Tree(3)),
new Tree(5, new Tree(6), new Tree(2)));
function printPreorder(tree) {
document.write("preorder:<br/>\n");
tree.preorder(function(v) { document.write(v + "<br/>\n"); });
}
printPreorder(myTree);
</script>
</body></html>
Implement the missing pieces so that the construction (new
Tree(...)) and iteration (tree.preorder(...)) at the
bottom of the script work, and produce the same output as the
equivalent Ruby and Python scripts above.
http://cs.nyu.edu/courses/summer12/CSCI-GA.3033-003/hw10.html